GitHub Actions is a powerful automation tool that can be used to build, test, and deploy software. By default, workflows are triggered by specific events, such as creating a pull request or pushing to a repository. However, there may be cases where you want to manually trigger a workflow. This is where the workflow_dispatch trigger comes in.
Using workflow_dispatch triggers, you can create workflows that can be manually triggered from the GitHub Actions UI or through the GitHub API. This can be useful in a number of scenarios:
- Testing: You can create a workflow that can be triggered manually to test new, or complex workflows, without having to perform more meaningful actions such as creating a pull request or creating a release.
- Flexibility: You can create a workflow that allows user input to control the execution of the workflow. This provides greater flexibility to your workflows and enables users to customize their workflows based on their needs.
- Reusability: You can create workflows that only need to run infrequently and not on any particular cadence. This enables teams to build reusable workflows that can be triggered manually; only as needed.
In this post, we’ll explore how to manually trigger GitHub Actions workflows using workflow_dispatch triggers. We’ll walk through the configuration of workflow_dispatch triggers and provide examples of how to define and use different workflow input types.
How to enable workflow_dispatch for a GitHub Actions workflow?
To enable workflow_dispatch triggers for a workflow, you need to add a workflow_dispatch
trigger to the on:
keyword in your workflow file.
name: Basic Workflow Dispatch Example on: workflow_dispatch: jobs: deploy: runs-on: ubuntu-latest steps: - name: Checkout uses: actions/checkout@v2

In this example, the workflow will only be run when manually triggered through the GitHub Actions UI or using the api.
The workflow must be checked into your default branch before you can trigger it the first time. However, once the workflow exists in the default branch, you can trigger it against any branch in your repository. This is quite helpful as it allows you to test out complex behaviors from another branch before having to check in to your default branch.
How to define inputs for workflow_dispatch workflows?
Once you’ve added the workflow_dispatch trigger to your workflow, you can define input types for the trigger. Input types allow you to capture user input and use it in your workflow.
Freeform input
In this example, we define an input called environment
that is required and has a default value of production
. This input can be used in your workflow using the ${{ github.event.inputs.environment }}
syntax or ${{ inputs.environment }}
syntax.
name: Basic Workflow Dispatch Example on: workflow_dispatch: inputs: environment: description: 'The environment to deploy to' required: true default: 'production' jobs: deploy: runs-on: ubuntu-latest steps: - name: Checkout uses: actions/checkout@v2 - name: Deploy run: echo "Deploying to ${{ github.event.inputs.environment }} environment"
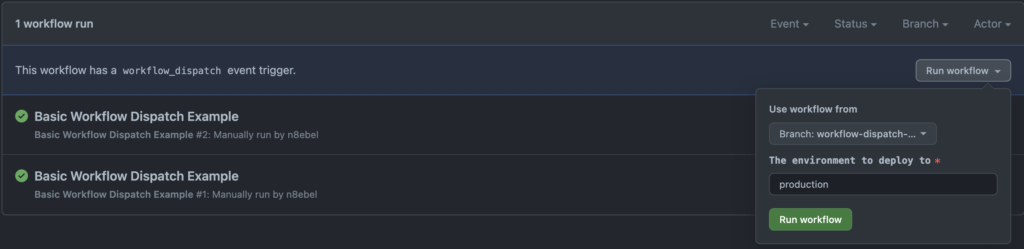
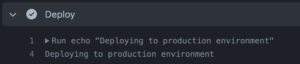
Constrained input sets
You can define input types that allow you to select from a constrained set of pre-defined options. This is particularly helpful for things like constraining which development environment to deploy to. In this example, we’ll define 3 possible options production
, qa
, and dev
with a default of dev
.
name: Basic Workflow Dispatch Example on: workflow_dispatch: inputs: environment: description: 'The environment to deploy to' required: true default: 'dev' type: choice options: - production - qa - dev jobs: deploy: runs-on: ubuntu-latest steps: - name: Checkout uses: actions/checkout@v2 - name: Deploy run: echo "Deploying to ${{ github.event.inputs.environment }} environment"
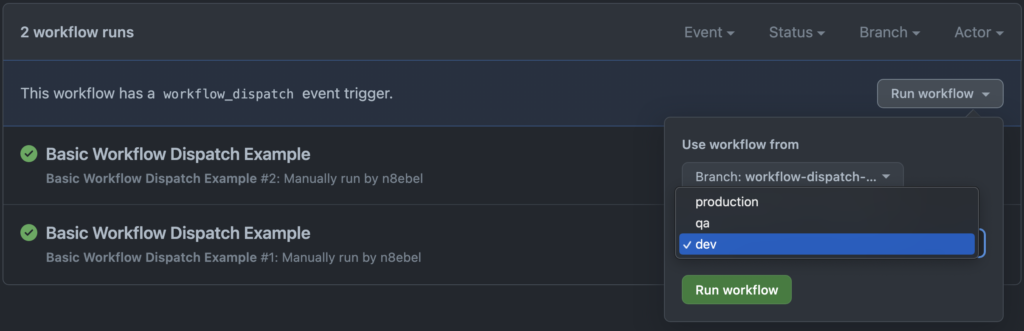
Multiple workflow inputs
A workflow_dispatch trigger can support multiple inputs at the same time; and we can mix-and-match input types. In the following example, we’ll combine a choice input type with a boolean input type to specify the deployment environment and to control whether or not a notification should be sent on completion of the workflow.
name: Basic Workflow Dispatch Example on: workflow_dispatch: inputs: environment: description: 'The environment to deploy to' required: true default: 'dev' type: choice options: - production - qa - dev notify: description: 'Should workflow notify team?' required: true type: boolean jobs: deploy: runs-on: ubuntu-latest steps: - name: Checkout uses: actions/checkout@v2 - name: Deploy run: echo "Deploying to ${{ github.event.inputs.environment }} environment" - name: Notify if: ${{ github.event.inputs.notify }} run: echo "Notifying of ${{ github.event.inputs.environment }} deployment"
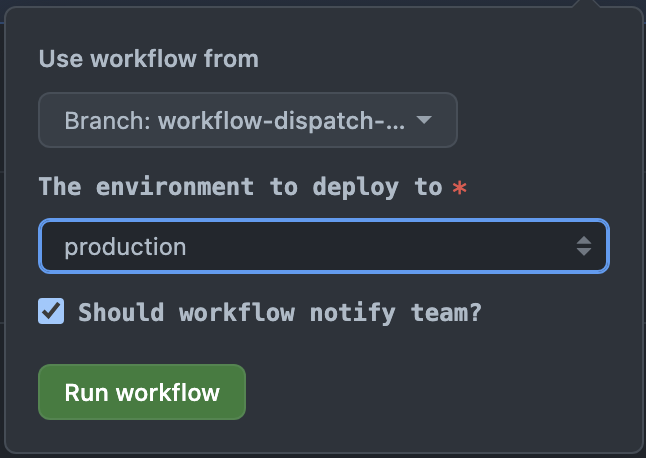
Wrapping Up
In conclusion, the workflow_dispatch trigger is a powerful feature in GitHub Actions that enables greater flexibility and control over the execution of workflows. It allows users to manually trigger workflows with customized inputs, making it easier to test and debug complex workflows. It also enables teams to build reusable workflows that only need to run infrequently, and not on any particular cadence. By following the examples and guidelines provided in this post, you can take advantage of the workflow_dispatch trigger and use it to enhance your GitHub Actions workflows.
Find other GitHub Actions posts here on goobar